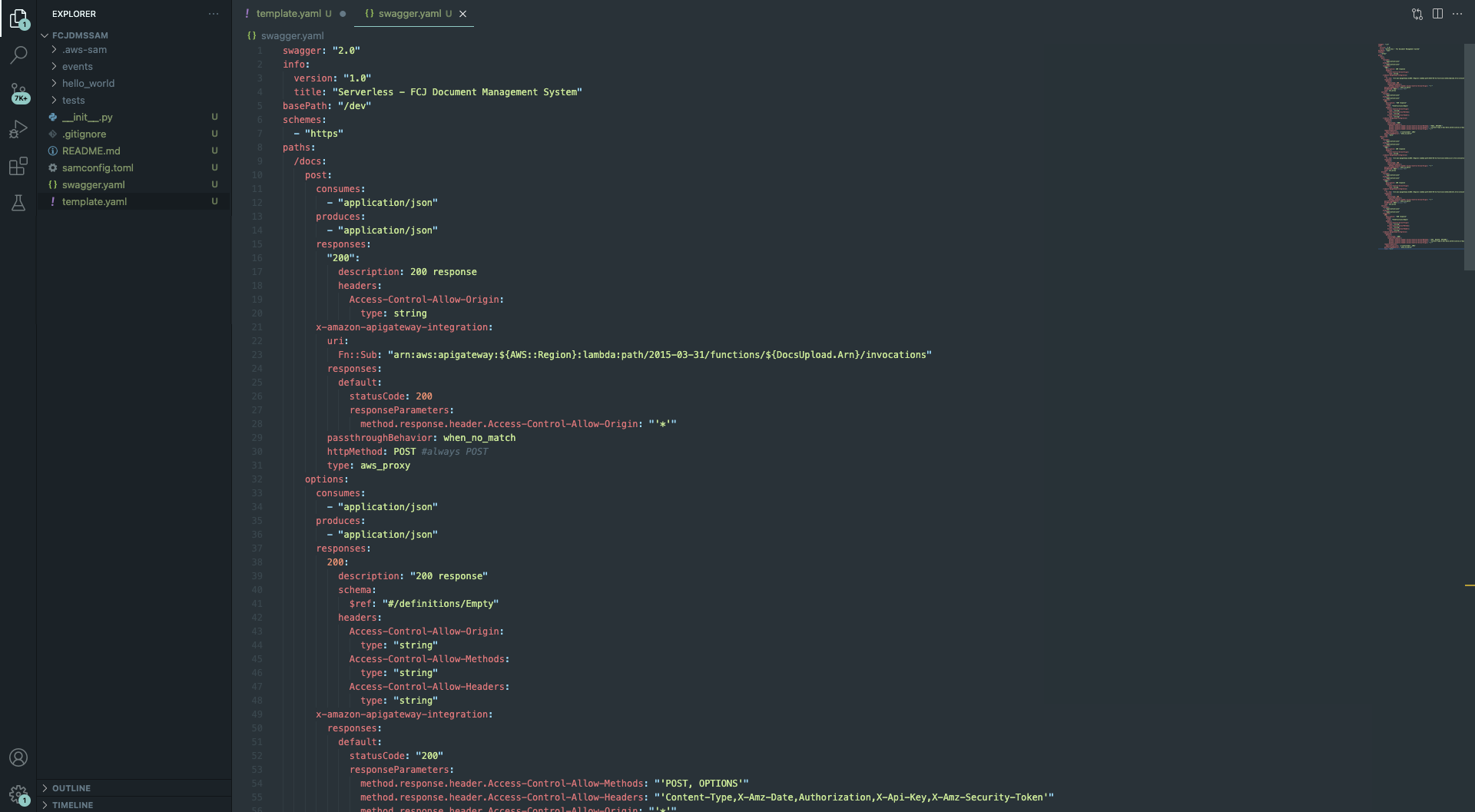
- Add the following code block to the bottom of template.yaml file to import swagger.yaml file.
# Create REST Api
DocApi:
Type: AWS::Serverless::Api
Properties:
StageName: dev
DefinitionBody:
'Fn::Transform':
Name: 'AWS::Include'
Parameters:
Location: "./swagger.yaml"
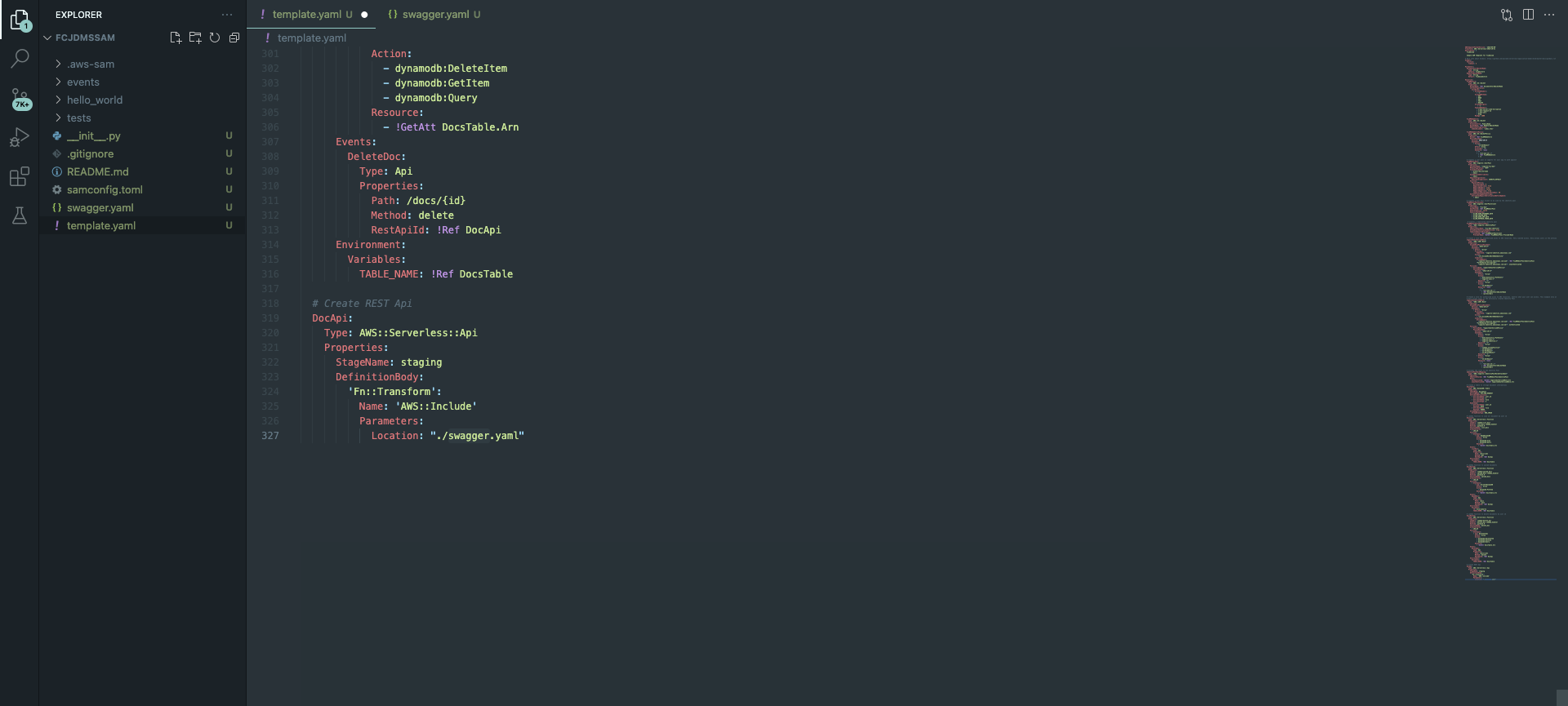
- Create folder and file: lambda/list_docs/list_docs.py
- Add the following code block to list_docs.py file
import json
import boto3
import os
from decimal import *
from boto3.dynamodb.types import TypeDeserializer
dynamodb = boto3.client('dynamodb')
serializer = TypeDeserializer()
class DecimalEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, Decimal):
return str(obj)
return json.JSONEncoder.default(self, obj)
def deserialize(data):
if isinstance(data, list):
return [deserialize(v) for v in data]
if isinstance(data, dict):
try:
return serializer.deserialize(data)
except TypeError:
return {k: deserialize(v) for k, v in data.items()}
else:
return data
def lambda_handler(event, context):
table_name = os.environ['TABLE_NAME']
user_id = event['pathParameters']['id']
print(user_id)
docs = dynamodb.query(
TableName=table_name,
KeyConditionExpression="user_id = :id",
ExpressionAttributeValues={ ":id": { 'S': user_id } }
)
format_data_docs = deserialize(docs["Items"])
# TODO implement
return {
"statusCode": 200,
"headers": {
"Content-Type": "application/json",
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Methods": "GET,PUT,POST,DELETE, OPTIONS",
"Access-Control-Allow-Headers": "Access-Control-Allow-Headers, Origin,Accept, X-Requested-With, Content-Type, Access-Control-Request-Method,X-Access-Token,XKey,Authorization"
},
"body": json.dumps(format_data_docs, cls=DecimalEncoder)
}
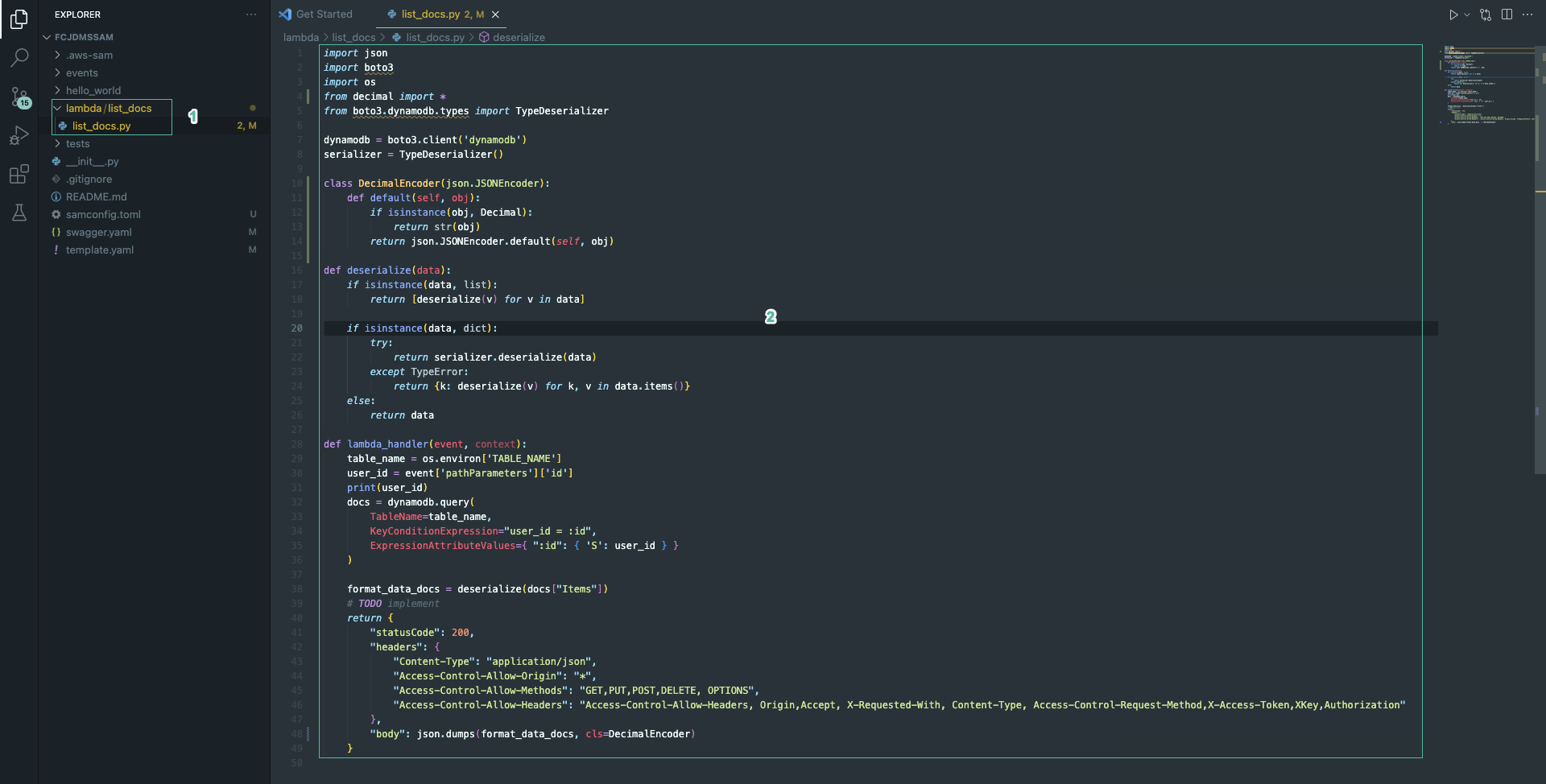
- Similarly, create upload_doc/upload_doc.py folder and file in lambda folder. Next copy the code below for the created file.
import json
import boto3
import os
from datetime import datetime, timezone
dynamodb = boto3.resource('dynamodb')
client_cloudwatch = boto3.client('cloudwatch')
def lambda_handler(event, context):
table_name = os.environ['TABLE_NAME']
now = datetime.now(tz=timezone.utc)
dt_string = now.strftime("%d/%m/%Y %H:%M:%S")
doc_data = json.loads(event["body"])
path = "protected/{}/{}".format(doc_data['identityId'], doc_data['file'])
doc_data.update({"path": path, "modified": dt_string})
table = dynamodb.Table(table_name)
table.put_item(Item = doc_data)
# TODO implement
return {
'statusCode': 200,
'body': 'successfully upload!',
'headers': {
'Content-Type': 'application/json',
"Access-Control-Allow-Headers": "Access-Control-Allow-Headers, Origin, Accept, X-Requested-With, Content-Type, Access-Control-Request-Method,X-Access-Token, XKey, Authorization",
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Methods": "GET,PUT,POST,DELETE,OPTIONS"
}
}
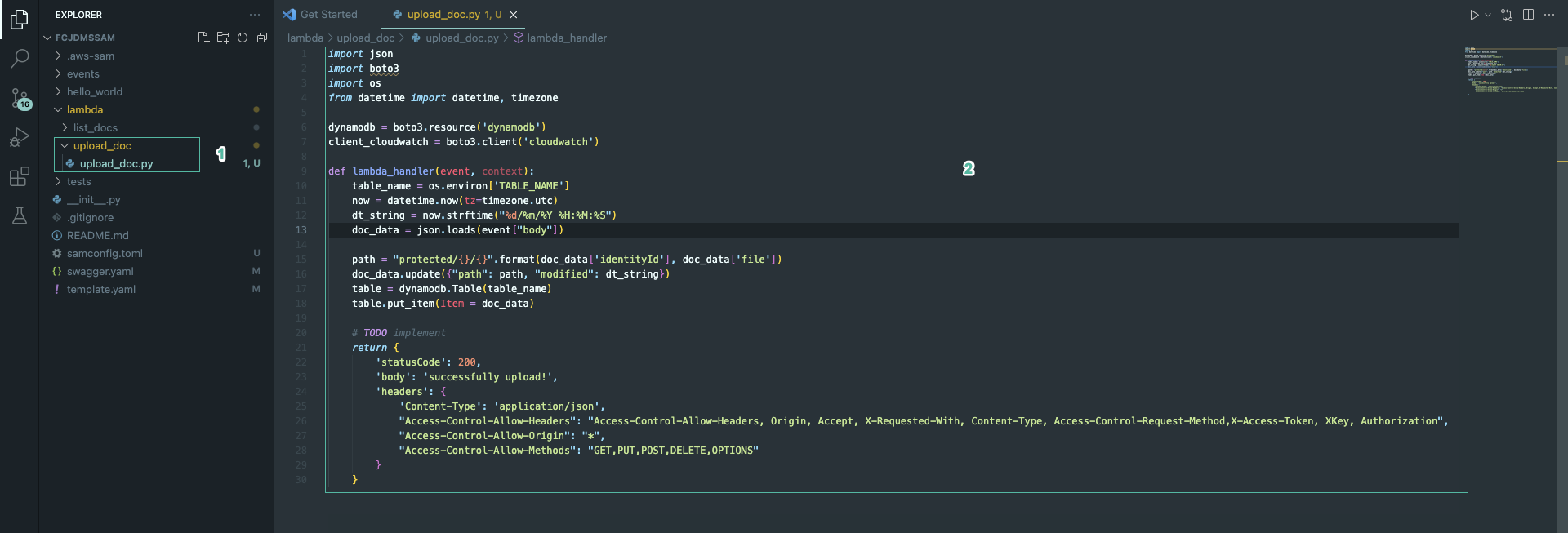
- Similar with lambda/delete_doc/delete_doc.py file.
import json
import boto3
import os
client = boto3.resource('dynamodb')
def lambda_handler(event, context):
# TODO implement
table_name = os.environ['TABLE_NAME']
error = None
doc_pk = event['pathParameters']['id']
print("doc_pk ", doc_pk)
doc_sk = event['queryStringParameters']['file']
print("doc_sk ", doc_sk)
table = client.Table(table_name)
key = {
'user_id':doc_pk,
'file': doc_sk
}
try:
table.delete_item(Key = key)
except Exception as e:
error = e
except Exception as e:
error = e
if error is None:
message = 'successfully document item!'
else:
print(error)
message = 'delete document fail'
return {
'statusCode': 200,
'body': message,
'headers': {
'Content-Type': 'application/json',
'Access-Control-Allow-Origin': '*'
},
}

- Similar with lambda/upload_general_infor/upload_general_infor.py file.
import json
import boto3
import os
dynamodb = boto3.resource('dynamodb')
def lambda_handler(event, context):
table_name = os.environ['TABLE_NAME']
data = json.loads(event["body"])
table = dynamodb.Table(table_name)
data.update({"id": event['pathParameters']['id']})
table.put_item(Item = data)
# TODO implement
return {
'statusCode': 200,
'body': 'successfully upload!',
'headers': {
'Content-Type': 'application/json',
"Access-Control-Allow-Headers": "Access-Control-Allow-Headers, Origin, Accept, X-Requested-With, Content-Type, Access-Control-Request-Method,X-Access-Token, XKey, Authorization",
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Methods": "GET,PUT,POST,DELETE,OPTIONS"
}
}
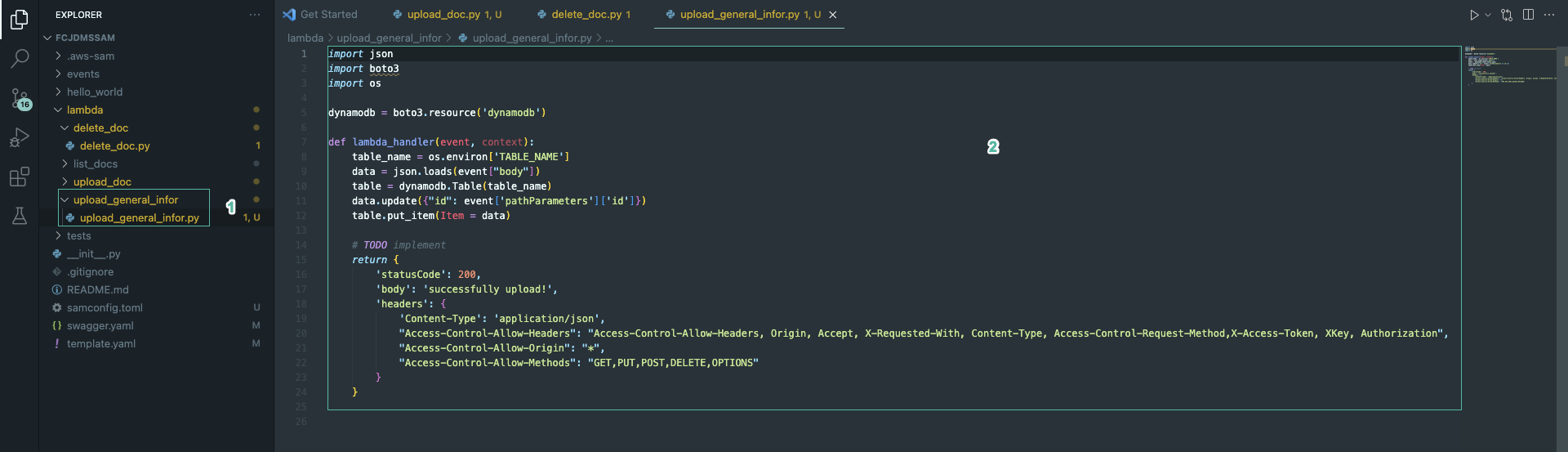
- Similar with lambda/get_general_infor/get_general_infor.py file.
import json
import boto3
import os
from decimal import *
from boto3.dynamodb.types import TypeDeserializer
dynamodb = boto3.client('dynamodb')
serializer = TypeDeserializer()
class DecimalEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, Decimal):
return str(obj)
return json.JSONEncoder.default(self, obj)
def deserialize(data):
if isinstance(data, list):
return [deserialize(v) for v in data]
if isinstance(data, dict):
try:
return serializer.deserialize(data)
except TypeError:
return {k: deserialize(v) for k, v in data.items()}
else:
return data
def lambda_handler(event, context):
# TODO implement
table_name = os.environ['TABLE_NAME']
user_id = event['pathParameters']['id']
print(user_id)
data = dynamodb.query(
TableName=table_name,
KeyConditionExpression="id = :id",
ExpressionAttributeValues={ ":id": { 'S': user_id } }
)
format_data = deserialize(data["Items"])
return {
"statusCode": 200,
"headers": {
"Content-Type": "application/json",
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Methods": "GET,PUT,POST,DELETE, OPTIONS",
"Access-Control-Allow-Headers": "Access-Control-Allow-Headers, Origin,Accept, X-Requested-With, Content-Type, Access-Control-Request-Method,X-Access-Token,XKey,Authorization"
},
"body": json.dumps(format_data, cls=DecimalEncoder)
#"body": format_data
}
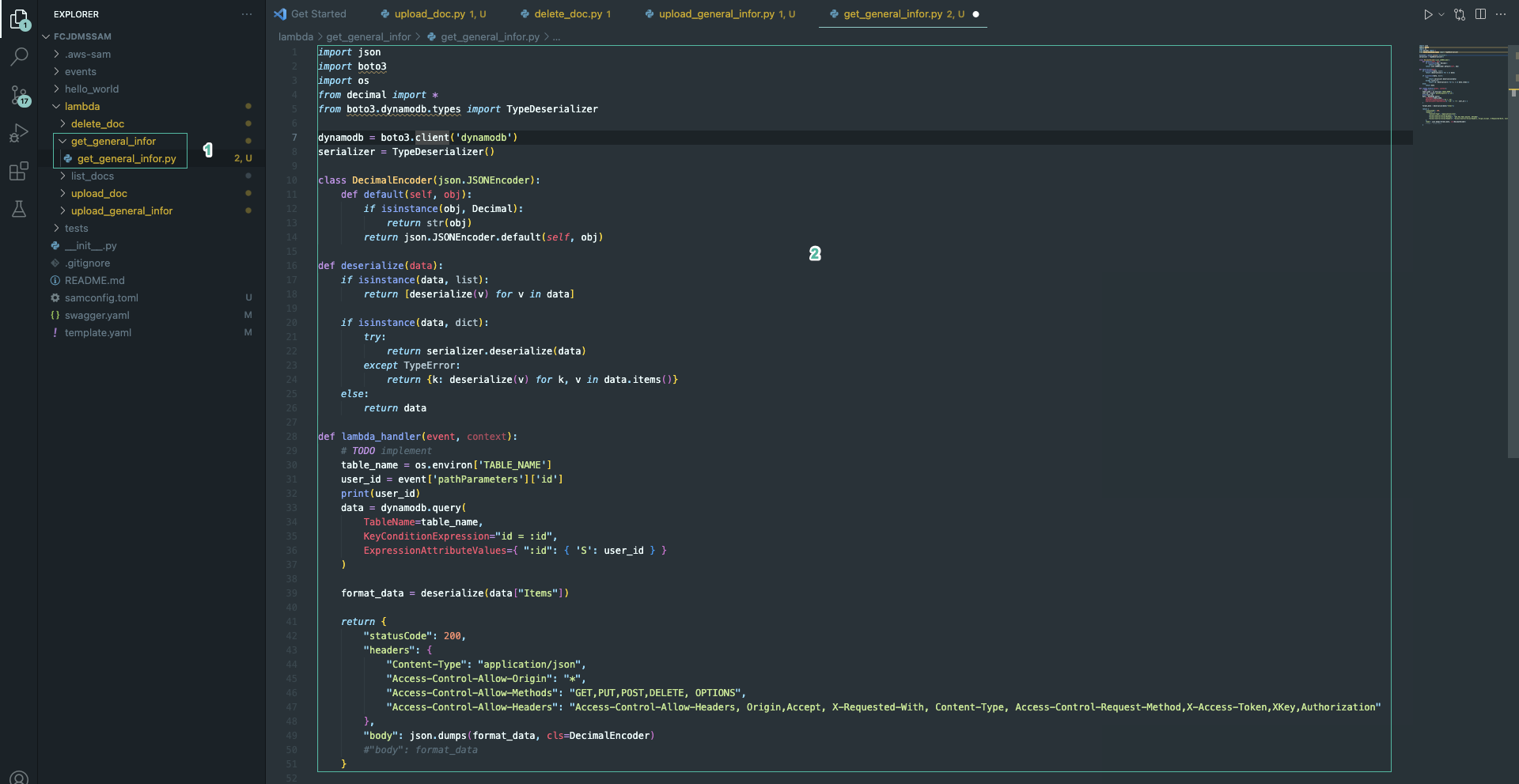
- Run the following command to build and deploy the sam project after updating:
sam build
sam deploy
While you wait for CloudFormation to complete, you can learn about the swagger.yaml file. The next part we will perform operations on the Front-end to test the operation of the APIs.